Optimizing Next.js Applications for Performance
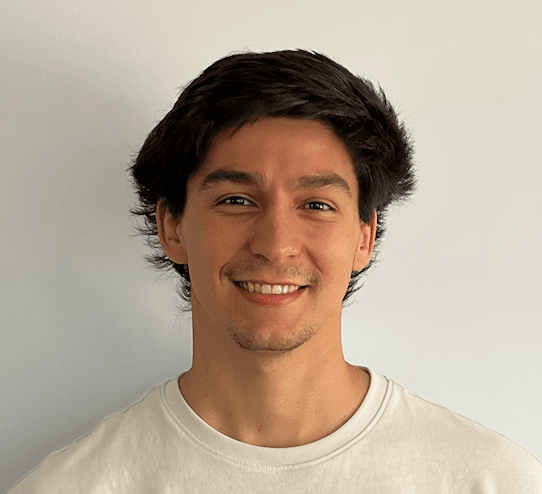
Bruno
5/20/2024

Introduction
Performance optimization is a critical aspect of web development that can significantly impact user experience and engagement. A fast and efficient application ensures that users have a smooth and responsive experience, which can lead to higher retention rates and better search engine rankings. In this article, we'll explore various techniques for optimizing Next.js applications, introduce some useful tools, and provide a real-world case study to demonstrate the effectiveness of these strategies.
Techniques
There are several techniques you can use to optimize the performance of your Next.js applications:
Code Splitting
Code splitting is a technique that allows you to split your code into smaller bundles that can be loaded on demand. This reduces the initial load time by only loading the code necessary for the current page. Next.js supports automatic code splitting, which means each page only loads what it needs.
Example: Dynamic Imports
Dynamic imports allow you to load components only when they are needed. This can significantly reduce the initial load time of your application.
// Example of dynamic import in Next.js
import dynamic from "next/dynamic";
const DynamicComponent = dynamic(
() => import("../components/DynamicComponent"),
{ ssr: false } // Disable server-side rendering for this component
);
// Usage in a page or another component
const Page = () => (
<div>
<h1>Hello, World!</h1>
<DynamicComponent />
</div>
);
export default Page;
By using dynamic imports, the DynamicComponent
will only be loaded when the user navigates to the page that uses it, reducing the initial bundle size.
Lazy Loading
Lazy loading is a strategy to defer the loading of non-critical resources at page load time. Instead, these resources are loaded when they are needed, such as when a user scrolls to a specific section of the page.
Example: Lazy Loading Images
Lazy loading images can drastically reduce the initial load time, especially on pages with many images.
// Example of lazy loading an image in Next.js
const LazyImage = () => (
<img src="/path/to/image.jpg" loading="lazy" alt="Description" />
);
You can also use the next/image
component for more advanced image optimization and lazy loading:
import Image from "next/image";
const OptimizedLazyImage = () => (
<Image
src="/path/to/image.jpg"
alt="Description"
width={800}
height={600}
layout="responsive"
loading="lazy"
/>
);
Image Optimization
Optimizing images is crucial for reducing load times and improving performance. Next.js provides a built-in Image
component that automatically optimizes images.
Example: Using the Next.js Image Component
The Image
component from Next.js handles image optimization out of the box, including resizing, lazy loading, and serving modern image formats like WebP.
import Image from "next/image";
const OptimizedImage = () => (
<Image
src="/path/to/image.jpg"
alt="Description"
width={800}
height={600}
layout="responsive"
loading="lazy"
/>
);
The layout="responsive"
attribute ensures that the image maintains its aspect ratio while adjusting to different screen sizes, improving the overall user experience.
Server Actions with Next.js 14
Server actions in Next.js 14 provide a more streamlined way to handle server-side operations. This can improve performance, especially for users with slower devices, by reducing the amount of JavaScript needed on the client side.
Example: Using Server Actions
Server actions allow you to fetch data on the server and pass it as props to your components, reducing the need for client-side data fetching.
// Example of server action in Next.js 14
export async function getServerSideProps() {
const data = await fetch("https://api.example.com/data").then((res) =>
res.json()
);
return { props: { data } };
}
const Page = ({ data }) => (
<div>
<h1>Server-side Rendered Data</h1>
<pre>{JSON.stringify(data, null, 2)}</pre>
</div>
);
export default Page;
By fetching data on the server, you can improve the initial load time and ensure that your application is SEO-friendly.
Tools
Several tools and libraries can help with performance optimization in Next.js:
- Lighthouse: An open-source tool from Google that helps you audit your web application's performance, accessibility, and SEO. Lighthouse provides a detailed report with suggestions on how to improve your site's performance.
- Webpack Bundle Analyzer: A tool that provides a visual representation of your bundle size, helping you identify large dependencies and optimize your bundle. This tool is particularly useful for pinpointing which parts of your codebase are contributing most to the bundle size.
- Next.js Analytics: A built-in analytics tool in Next.js that helps you monitor and improve the performance of your application. It provides insights into various performance metrics and helps you identify potential bottlenecks.
Using Lighthouse
You can use Lighthouse directly from Chrome DevTools to analyze your application:
- Open your application in Google Chrome.
- Open DevTools (right-click on the page and select "Inspect" or press
Ctrl+Shift+I
). - Navigate to the "Lighthouse" tab.
- Click "Generate report" to run the audit.
Lighthouse will provide you with a detailed report on your application's performance, accessibility, best practices, and SEO, along with suggestions for improvements.
Using Webpack Bundle Analyzer
To use Webpack Bundle Analyzer with Next.js, you can install the package and modify your next.config.js
:
npm install @next/bundle-analyzer
// next.config.js
const withBundleAnalyzer = require('@next/bundle-analyzer')({
enabled: process.env.ANALYZE === 'true',
});
module.exports = withBundleAnalyzer({});
Then, you can run the analyzer with the following command:
ANALYZE=true npm run build
This will generate a visual representation of your bundle, helping you identify large dependencies.
Case Study
Let's take a look at a real-world example of optimizing a Next.js application. Imagine you have an e-commerce site built with Next.js, and you're noticing high bounce rates and slow load times. Here's how you could optimize it:
-
Analyze Performance: Use Lighthouse to audit the current performance of your site and identify areas for improvement. Pay attention to metrics like First Contentful Paint (FCP), Largest Contentful Paint (LCP), and Time to Interactive (TTI).
-
Implement Code Splitting: Refactor your code to use dynamic imports and reduce the initial load time. For example, you can dynamically import components that are not critical for the initial render:
import dynamic from 'next/dynamic'; const Cart = dynamic(() => import('../components/Cart'), { ssr: false, }); const HomePage = () => ( <div> <h1>Welcome to Our Store</h1> <Cart /> </div> ); export default HomePage;
-
Enable Lazy Loading: Update your images and other non-critical resources to use lazy loading. This ensures that only images in the viewport are loaded initially:
const ProductImage = ({ src, alt }) => ( <img src={src} alt={alt} loading="lazy" /> );
-
Optimize Images: Replace standard image elements with the Next.js
Image
component to ensure all images are optimized:
import Image from 'next/image';
const ProductImage = ({ src, alt }) => (
<Image src={src} alt={alt} width={500} height={500} layout="responsive" />
);
-
Switch to Server Actions: Convert static pages to use server actions to improve performance for users with slower devices. For instance, fetch product data on the server:
export async function getServerSideProps() { const products = await fetch('https://api.example.com/products').then(res => res.json()); return { props: { products } }; } const ProductsPage = ({ products }) => ( <div> <h1>Our Products</h1> <ul> {products.map(product => ( <li key={product.id}>{product.name}</li> ))} </ul> </div> ); export default ProductsPage;
After implementing these optimizations, you re-run the Lighthouse audit and see a significant improvement in performance scores, reduced bounce rates, and higher user engagement.
Conclusion
Optimizing the performance of your Next.js applications is essential for providing a fast and seamless user experience. By using techniques such as code splitting, lazy loading, image optimization, and server actions in Next.js 14, you can significantly improve the performance of your applications. Utilize tools like Lighthouse, Webpack Bundle Analyzer, and Next.js Analytics to monitor and enhance your site's performance continuously.
Explore our platform's extensive library of Next.js templates, which are designed with performance in mind, and use our filters to find the best templates to kickstart your project. Remember, a well-optimized application not only improves user satisfaction but also boosts your SEO rankings and overall success. Happy coding!