Best Practices for Maintaining and Updating Next.js Projects
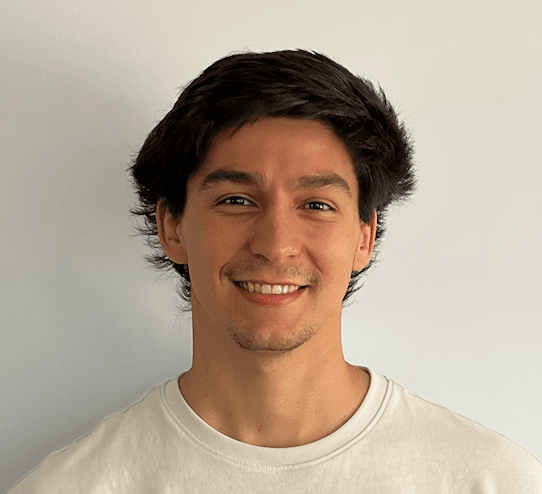
Bruno
5/27/2024

Introduction
Maintaining and updating your Next.js projects is crucial for ensuring they remain secure, performant, and up-to-date with the latest features. Proper maintenance practices help you avoid technical debt, reduce bugs, and improve the overall quality of your codebase. In this guide, we'll discuss best practices for maintaining and updating Next.js projects, including version control, dependency management, testing, and regular updates.
Version Control
Version control systems, like Git, are essential tools for managing changes to your codebase. They enable you to track changes, collaborate with other developers, and revert to previous versions if needed.
Using Git for Version Control
-
Initialize a Git Repository: If you haven't already, initialize a Git repository in your project directory:
git init
-
Create a .gitignore File: Add a
.gitignore
file to exclude files and directories that you don't want to track. For a Next.js project, a typical.gitignore
file might include:node_modules/ .next/ .env.local .env.development.local .env.test.local .env.production.local
-
Commit Changes Regularly: Commit your changes frequently with descriptive commit messages:
git add . git commit -m "Add initial project setup"
-
Use Branches for Features and Fixes: Create separate branches for new features and bug fixes to keep your main branch stable:
git checkout -b feature/new-feature
-
Merge Changes and Resolve Conflicts: When your feature or fix is ready, merge it back into the main branch and resolve any conflicts that arise:
git checkout main git merge feature/new-feature
Dependency Management
Managing dependencies is a critical aspect of maintaining your Next.js projects. Keeping dependencies up to date ensures that your project benefits from the latest features, bug fixes, and security patches.
Tips for Managing Dependencies
-
Use a Package Manager: Use npm or yarn to manage your project's dependencies. For example, to install a package with npm:
npm install package-name
-
Check for Updates Regularly: Regularly check for outdated packages and update them. You can use tools like
npm outdated
to see which packages need updating:npm outdated
-
Update Dependencies: Update your dependencies to their latest versions:
npm update
-
Use Dependabot or Renovate: Automate dependency updates by integrating tools like Dependabot or Renovate into your GitHub repository. These tools create pull requests to update your dependencies, making it easier to keep your project up to date.
-
Test After Updates: Always test your project thoroughly after updating dependencies to ensure that nothing is broken and that the updates don't introduce new bugs.
Testing
Testing is crucial for maintaining the quality and reliability of your Next.js projects. It helps you catch bugs early, ensures your code behaves as expected, and provides confidence when making changes.
Recommended Testing Frameworks for Next.js
-
Jest: Jest is a popular testing framework for JavaScript applications. It provides a comprehensive testing solution, including unit tests, integration tests, and snapshot tests.
npm install --save-dev jest
-
React Testing Library: React Testing Library is a testing library specifically designed for testing React components. It encourages testing practices that resemble how users interact with your application.
npm install --save-dev @testing-library/react
-
Cypress: Cypress is an end-to-end testing framework that allows you to test your application in a real browser environment.
npm install --save-dev cypress
Writing Tests
-
Unit Tests: Write unit tests to test individual components and functions. For example, using Jest and React Testing Library:
// components/Header.js export default function Header() { return <h1>Hello, World!</h1>; } // components/Header.test.js import { render, screen } from '@testing-library/react'; import Header from './Header'; test('renders header', () => { render(<Header />); expect(screen.getByText('Hello, World!')).toBeInTheDocument(); });
-
Integration Tests: Write integration tests to test how different parts of your application work together.
-
End-to-End Tests: Write end-to-end tests using Cypress to test the entire flow of your application from the user's perspective.
// cypress/integration/home.spec.js describe('Home Page', () => { it('should display the welcome message', () => { cy.visit('/'); cy.contains('Welcome to My Website'); }); });
Regular Updates
Keeping your Next.js framework and other libraries up to date is essential for maintaining a secure and efficient codebase.
Advice on Regular Updates
-
Monitor Releases: Keep an eye on the release notes for Next.js and other key libraries you use. Subscribe to newsletters or follow relevant GitHub repositories to stay informed about new releases and updates.
-
Update the Next.js Framework: Regularly update Next.js to benefit from the latest features, performance improvements, and security patches.
npm install next@latest
-
Perform Regular Code Reviews: Regularly review your codebase to identify areas that might need refactoring or updates. Code reviews help maintain code quality and ensure adherence to best practices.
-
Use Continuous Integration (CI): Integrate a CI pipeline to automate testing and deployment. Tools like GitHub Actions, CircleCI, or Travis CI can help you automate these processes, ensuring that your code is always tested and deployed correctly.
// Example GitHub Actions workflow file: .github/workflows/ci.yml name: CI on: [push] jobs: build: runs-on: ubuntu-latest steps: - uses: actions/checkout@v2 - name: Set up Node.js uses: actions/setup-node@v2 with: node-version: '14' - run: npm install - run: npm test - run: npm run build
Conclusion
Maintaining and updating your Next.js projects is vital for ensuring their longevity, security, and performance. By following best practices for version control, dependency management, testing, and regular updates, you can keep your projects running smoothly and efficiently. Utilizing templates from our directory can further streamline your development process, allowing you to focus on building high-quality applications.
Explore our platform's extensive library of Next.js templates to find the perfect starting point for your next project. Remember, regular maintenance and updates are key to the success of any software project. Keep your projects up to date, and happy coding!