Getting Started with Next.js. A Beginner's Guide
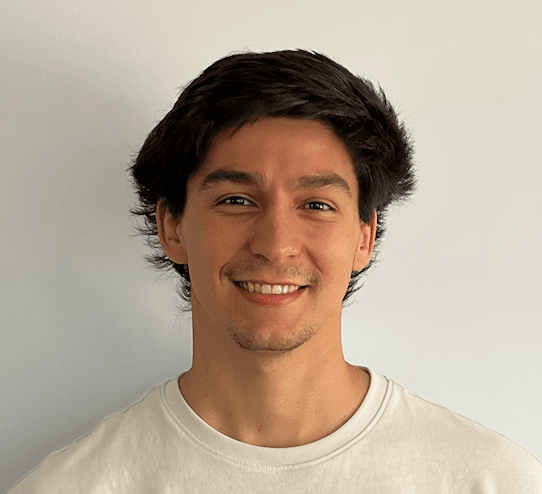
Bruno
5/27/2024
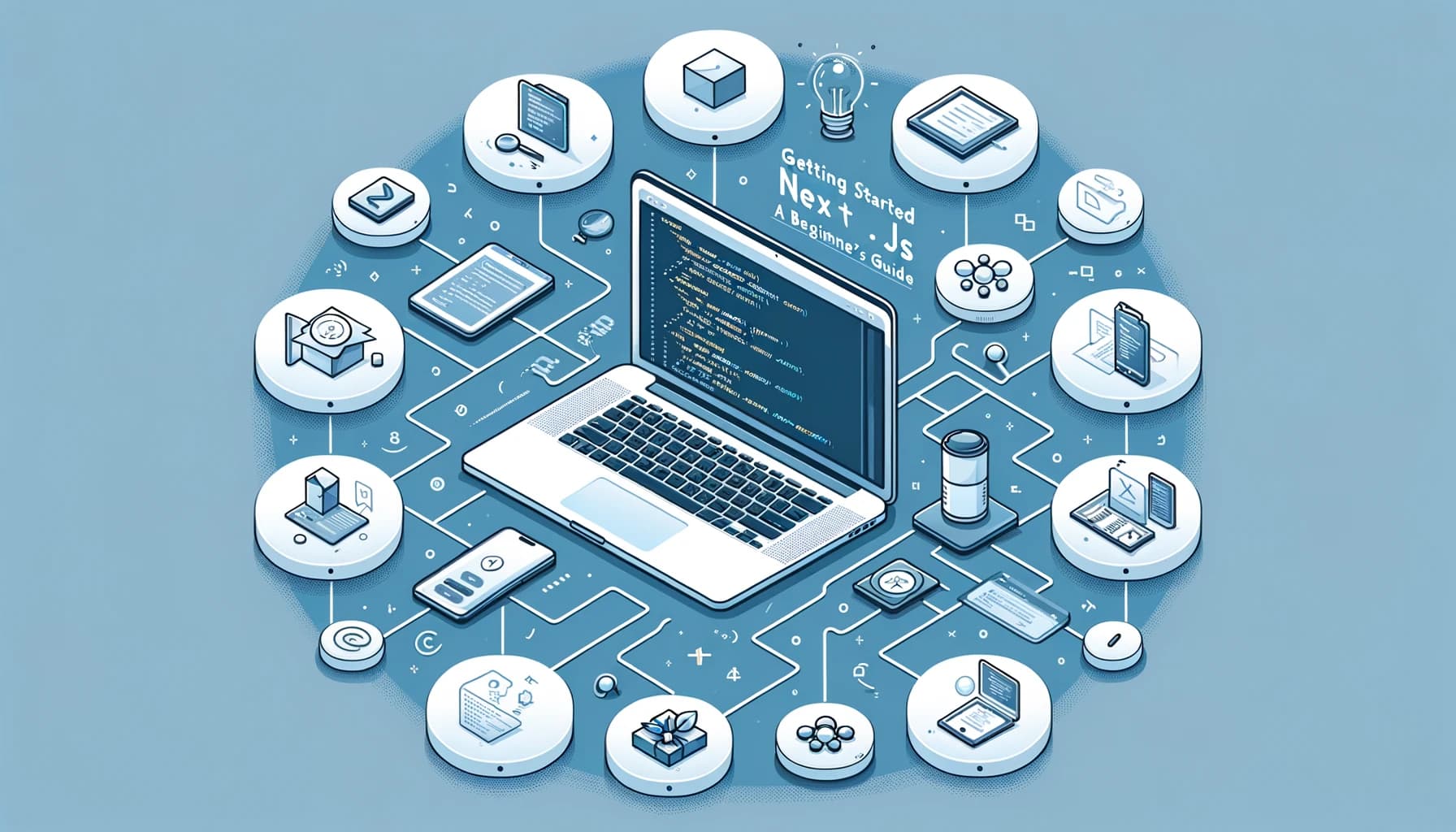
Introduction
Next.js is a powerful React framework that enables developers to build fast and user-friendly static websites and web applications. It offers a range of features that simplify the development process, including server-side rendering, static site generation, API routes, and the latest additions like the App Router and Server Actions. By combining the power of React with these advanced capabilities, Next.js helps developers create high-performance applications with ease.
In this beginner's guide, we'll walk you through the basics of getting started with Next.js 14. You'll learn how to set up your development environment, understand the core concepts, and use templates to jumpstart your projects. Whether you're a seasoned developer or just starting out, this guide will provide you with the foundational knowledge you need to succeed with Next.js 14.
Setup
Setting up a Next.js development environment is straightforward. Follow these steps to get started:
-
Install Node.js and npm: Next.js requires Node.js and npm (Node Package Manager). You can download and install them from the official Node.js website.
-
Create a New Next.js Project: Once Node.js and npm are installed, you can create a new Next.js project using the following command:
npx create-next-app@latest my-nextjs-app
This command will prompt you to enter a project name and select various options for your project setup. Once completed, it will generate a new Next.js project with the specified name.
-
Navigate to Your Project Directory: Change into your project directory using the
cd
command:cd my-nextjs-app
-
Start the Development Server: Start the Next.js development server with the following command:
npm run dev
This will start the development server, and you can view your new Next.js application by opening a browser and navigating to
http://localhost:3000
.
Congratulations! You have successfully set up your Next.js development environment and started your first Next.js project.
Basic Concepts
To build efficient applications with Next.js 14, it's essential to understand its core concepts. Let's explore some of the key concepts that form the foundation of Next.js:
App Router
The App Router in Next.js 14 allows you to define routes using the file system. Each file in the app
directory represents a different route, and the folder structure determines the routing hierarchy.
Example: Creating a New Page
Create a new folder called about
inside the app
directory, and add a file named page.js
:
app/
├── page.js
├── about/
├── page.js
Add the following code to app/about/page.js
:
export default function AboutPage() {
return (
<div>
<h1>About Us</h1>
<p>Welcome to the About Us page.</p>
</div>
);
}
This will create a new page accessible at http://localhost:3000/about
.
Layouts and Nested Routing
Next.js 14 introduces layouts and nested routing, allowing you to create reusable layout components.
Example: Creating a Layout
Create a layout file in the app
directory:
app/
├── layout.js
├── page.js
├── about/
├── page.js
Add the following code to app/layout.js
:
export default function RootLayout({ children }) {
return (
<html>
<body>
<header>
<h1>My Website</h1>
<nav>
<a href="/">Home</a>
<a href="/about">About</a>
</nav>
</header>
<main>{children}</main>
</body>
</html>
);
}
This layout will wrap all pages within the app
directory, providing a consistent structure.
Server Actions
Server Actions in Next.js 14 provide a way to handle server-side operations more efficiently. They allow you to fetch data and perform server-side logic directly in your components.
Example: Using Server Actions
Create a new file called serverActions.js
in the app
directory:
app/
├── page.js
├── about/
├── page.js
├── serverActions.js
Add the following code to app/serverActions.js
:
export async function getData() {
const res = await fetch('https://api.example.com/data');
const data = await res.json();
return data;
}
You can now use this server action in your page components:
import { getData } from './serverActions';
export default async function HomePage() {
const data = await getData();
return (
<div>
<h1>Data from Server</h1>
<pre>{JSON.stringify(data, null, 2)}</pre>
</div>
);
}
This approach allows you to handle data fetching and server-side logic seamlessly within your components.
Using Templates
Next.js templates can help you get started quickly by providing a solid foundation for your project. Our template directory offers a variety of templates tailored to different types of applications.
Example: Using a Template
-
Choose a Template: Browse through our template directory and choose a template that fits your project needs.
-
Download the Template: Follow the instructions provided to download and set up the template.
-
Customize the Template: Modify the template to match your project requirements. This might involve updating the content, adding new pages, and customizing the styles.
For example, if you choose a blog template, you might find a structure like this:
app/
├── layout.js
├── page.js
├── posts/
├── [slug].js
├── components/
├── Layout.js
├── Post.js
You can customize the Layout.js
and Post.js
components to fit your design preferences, and add new posts in the posts
directory.
Conclusion
Getting started with Next.js 14 is an exciting journey that opens up a world of possibilities for building fast, efficient, and scalable web applications. By understanding the core concepts of the App Router, layouts, and Server Actions, you can create powerful applications with ease. Using templates from our directory can further accelerate your development process, allowing you to focus on building features that matter.
Next.js 14 continues to evolve, offering new features and improvements that make it a top choice for developers. To stay updated and expand your knowledge, explore the official Next.js documentation and join the vibrant Next.js community.
Happy coding!